What is Storybook? (Part 1)
Nowadays, people are used to working with applications with an intuitive, seamless user interface. An intuitive interface works the way users expect it to. It means users don’t have to think about how to reach their goals, for example, creating an account. Therefore, applications won’t make it if the UX/UI doesn’t match their expectations.
Storybook helps you to focus on developing user interfaces without dealing with business logic and implementing these in an application. It’s an open-source web-based tool that creates a development environment to develop UI components, layouts, and pages in isolation without dealing with business logic and implementing these in an application.
It enables you to focus on component states and edge cases that are difficult to reproduce when developing without using Storybook. An important thing to mention is that it integrates with frameworks like React, Angular, Vue, and more.
Why use Storybook?
A design system is a set of standards to manage design at scale by reducing redundancy while creating a shared language and visual consistency across different pages and channels.
Designs are more complex, and the front-end has more responsibilities than it used to. You need to think about consistency, performance, and accessibility during development. A Design system is a set of standards to manage design to keep user interfaces consistent. If you care about usability and production, testing every component is good practice before going to production. Storybook helps you to handle these challenges.
Translating designs into robust components, layouts, and pages that cover all application use cases is challenging. That comes with their different states that create an intuitive user interface. For example, it could be hard to keep track of all these states during development, especially for the designers and other stakeholders who want to review these components. Wouldn’t it be nice for UX designers to test their ideas with users before implementing them in the main application?
Storybook is an excellent tool to accomplish this. It could significantly improve the development flow of an enterprise application with complex UX. It has benefits for developers, UX designers, and other stakeholders:
- Making documentation easy for components;
- It gives developers the tooling to develop in isolation outside an existing application;
- Enables collaboration between developers, UX designers, and other stakeholders during the development;
- Designers can validate components early in the process;
- Creates an overview of all states and edge cases in different viewports like mobile, tablet, and desktop;
- Helping with testing new user interactions before implementation in a production application;
- Increases developer awareness of existing components in the application.
All these benefits contribute to robust components and better communication between developers, designers, and other stakeholders.
The disadvantages of using Storybook
Storybook has its downsides. To use it in your development workflow, you will have to commit to it. Use Storybook for all components within your application landscape, or it becomes unclear which components exist in Storybook and which don’t.
Maintaining your Storybook is very important, and it’s easy to forget it when it’s not a part of your way of working. When you add something new to a component, you must check Storybook, update the stories, and deploy the latest version. Using Storybook comes with a price, but all the work pays off in the end.
An example of how to use Storybook to create a button in React
When the UX designer of your team comes up with a button with the following requirements: “the button needs one or two text lines and has the states: default and primary.” You can create the button in your application or use Storybook to develop the button, and have an overview with all possible variations with the advantage that you can control all the props of the button. For example, change the button's text and replace it with a big word or sentence to determine if the text is rendering correctly.
First, you create the Button component with an interface so it can handle the requirements.
import "./Button.css";
interface ButtonProps {
children: string;
subtext?: string;
primary?: boolean;
}
export function Button({ subtext, primary = false, children }: ButtonProps) {
return (
<button className={primary ? "primary" : ""}>
{children} {subtext &amp;&amp; <span className="subtext">{subtext}</span>}
</button>
);
}
Secondly, you create a story file Button.stories.tsx
with all the stories to
describe the state of the button. These stories are used in Storybook to render
the button component with the arguments as component properties.
// Button.stories.tsx
// import Meta and Story interfaces
import type { Meta, Story } from "@storybook/react";
// import ExampleComponent
import { Button } from "./Button";
// export default Story metadata
export default {
title: ‘Button’, // Title of story
component: Button, // main component used in the story
} as Meta<typeof Button>;
const Template: Story<typeof Button> = (args) => <Button {...args} />; // template to creating stories
// default button
const Default = Template.bind({});
Default.args = {
children: "Button text",
};
// primary button
const Primary = Template.bind({});
Primary.args = {
children: "Button text",
primary: true
};
// default button with subtext
const WithSubtext = Template.bind({});
WithSubtext.args = {
children: "Button text",
subtext: “It’s free”
};
Finally, you can see the stories in Storybook when you run npm run storybook
.
You can deploy Storybook on Github pages so designers and other stakeholders
access the stories to review. See
https://rkonings.github.io/storybook-button-example
for an example.
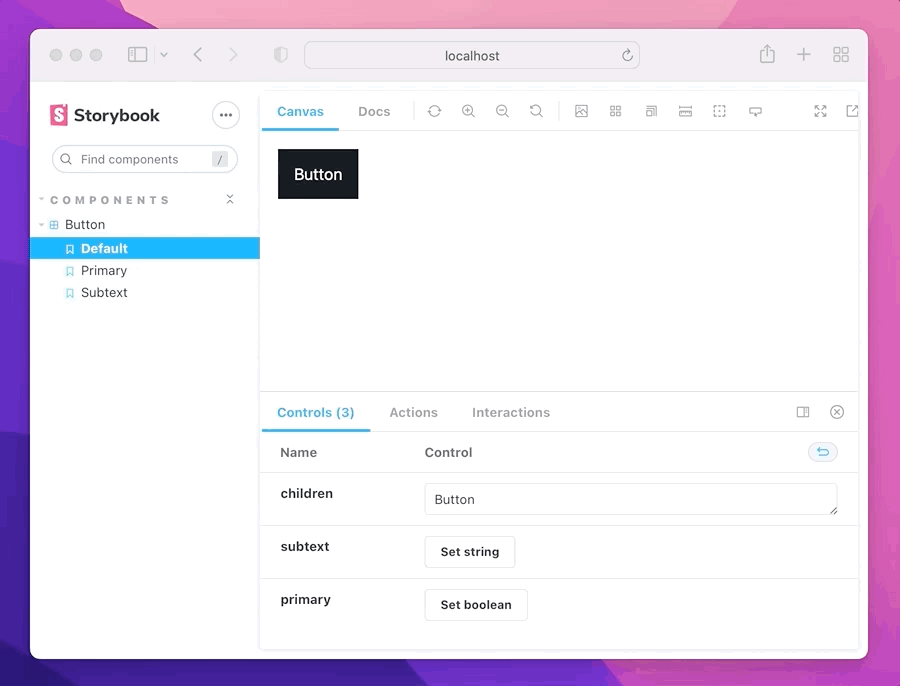
Is Storybook something for your project?
It can feel time-consuming when you start with Storybook. Developing components in isolation and not in the main application looks like overengineering. I promise you it becomes much easier after a couple of components. Especially in an enterprise application, Storybook helps to bring an application to the next level.
My advice would be to use Storybook to develop a new feature, create your components in Storybook before implementing them in an application, and finally deploy Storybook so designers and other stakeholders can see the progress and test it with users. In addition, you will have robust components and an overview of all the states and edge cases.
It will have a positive effect on collaboration between designers, developers, and other stakeholders and will significantly improve the transparency of your team's way of working.
I hope you are inspired to try Storybook in your project. In my next article, I will discuss my lessons learned using Storybook in an enterprise application.