Stop writing boilerplate code in IntelliJ
Did you ever work in a codebase with a lot of boilerplate code? Repetitive stuff that just needs to be there. The brainless kind of copy-paste work. I did. And you probably did as well.I'll give you an example. I worked in a codebase that required a singleton pattern for almost all of the classes. The singleton class looked like this:
export class ProductService {
private static instance: ProductService;
private constructor(
private readonly cartService: CartService,
private readonly httpClient: HttpClient
) { }
static create(cartService: CartService, httpClient: HttpClient): ProductService {
if (!ProductService.instance) {
ProductService.instance = new ProductService(cartService, httpClient);
}
return ProductService.instance;
}
static singleton(): ProductService {
if (!ProductService.instance) throw new Error('ProductService.singleton was called before initialization');
return ProductService.instance;
}
... // instance methods
}
You can imagine that writing all of this out everytime is really time consuming, not much fun and also error prone.
One way to deal with this, is to simply copy-paste other classes and edit them to your needs. This reduces the error-rate a bit, but still takes time. Luckily, there is a faster way to deal with this.
IntelliJ live templates
We can create a code snippet to handle writing the boilerplate code for us. In IntelliJ, these code snippets are called 'Live templates'. IntelliJ already provides us with a lot of code snippets. They can be found here:
- Open settings (cmnd + ,)
- navigate to Editor -> Live templates
It is definitely worth it to go through the list.
Of course, we can create our own code snippets as well. Inside the Live templates menu, select the language/framework you want to add your code snippet to. Then press the '+'-sign to add one.
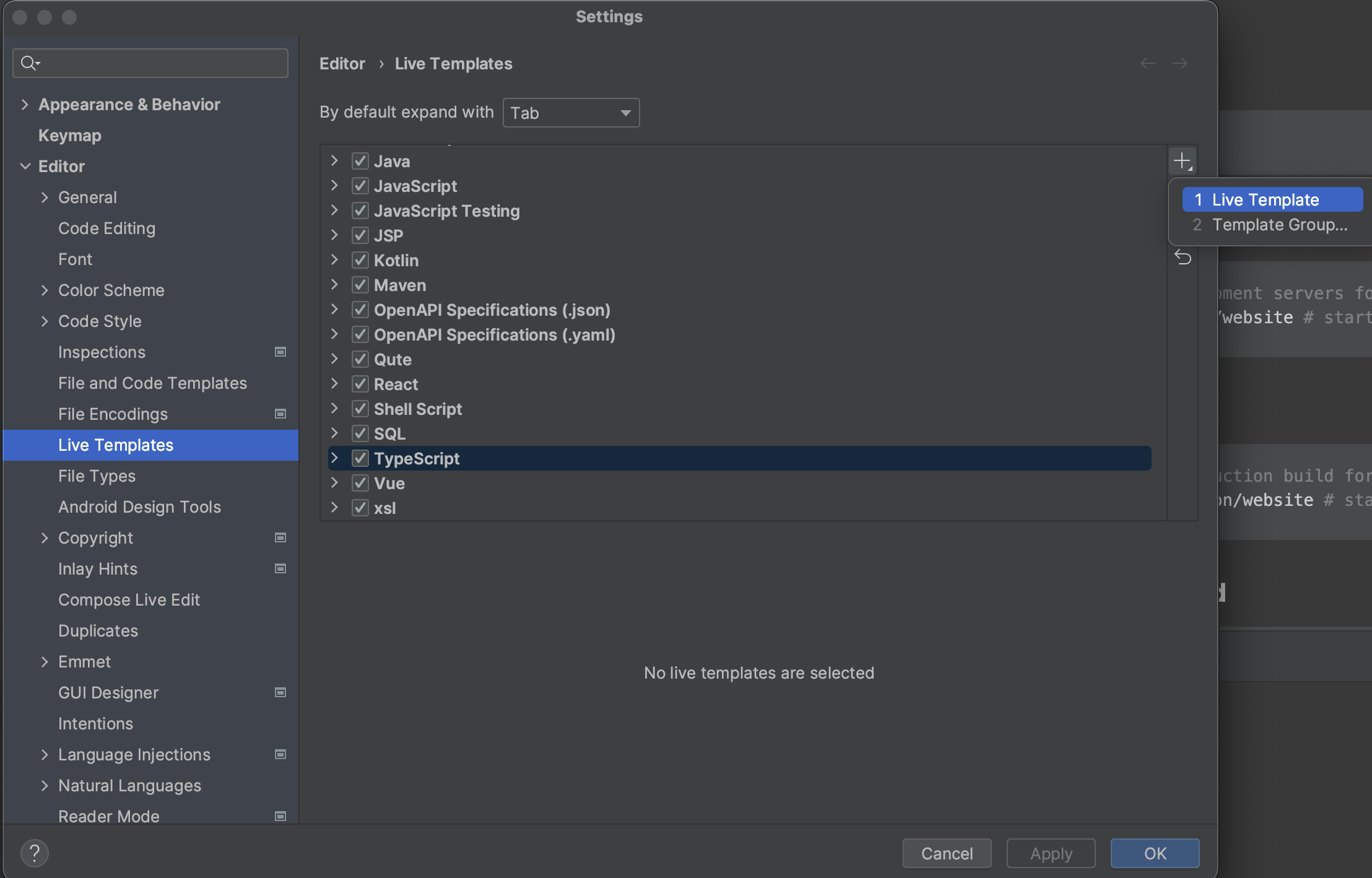
You can define an abbreviation (ie. singletondep) and an optional description. Not all code snippets are available everywhere. This is why you need to define the context of your code snippet. Use the 'Define' dropdownlist to define the context. YOUR CODESNIPPET WILL NOT WORK IF YOU SKIP THIS.
Lastly, the template text. This is where the magic happens, because you can work with variables here. Let's say that we want to create a code snippet for our Singleton without dependencies. We can define it as follows:
export class $NAME$ {
private static instance: $NAME$;
private constructor(private readonly $DEP_NAME$: $DEP_TYPE$) {}
static create($DEP_NAME$: $DEP_TYPE$): $NAME$ {
if (!$NAME$.instance) {
$NAME$.instance = new $NAME$($DEP_NAME$);
}
return $NAME$.instance;
}
static singleton(): $NAME$ {
if (!$NAME$.instance) throw new Error('$NAME$.singleton was called before it was created');
return $NAME$.instance;
}
}
So, now you can create a typescript file, type 'singetondep', press 'tab' and IntelliJ will insert your code snippet.
We have 3 variables here: $NAME$
, $DEP_NAME$
and $DEP_TYPE$
. After inserting the code snippet, you will be prompted
to enter the variables one by one. Press 'enter' after each value. See the example below.
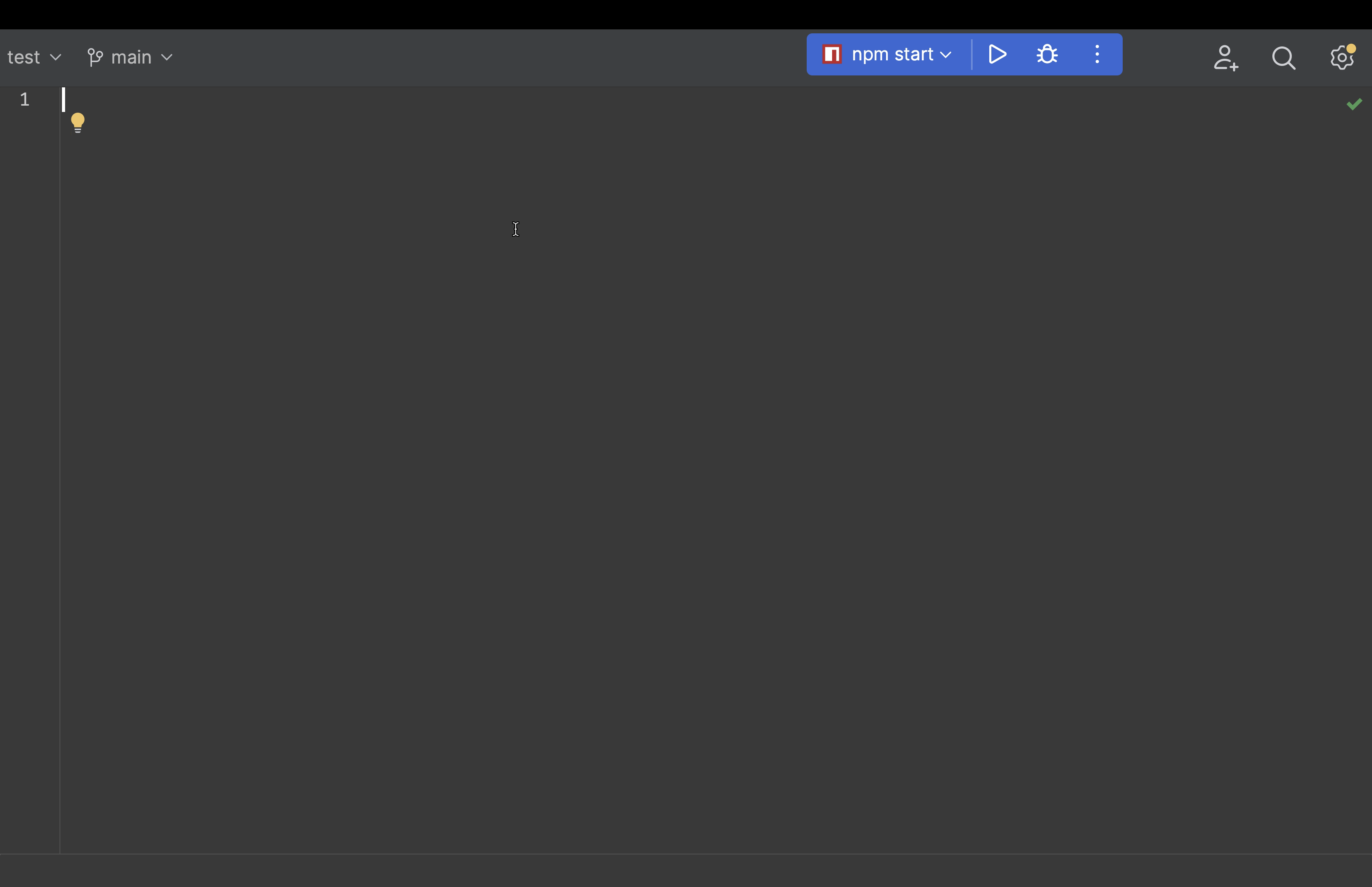
Existing snippets
I also particularly like the JavaScript Testing code snippets. I use them a lot, although the default settings for these snippets aren't quite up to date. If you'd check out the default code snippets for the describe function (abbreviation: 'descr'), you'll see the old 'function' keyword being used where an arrow function is more appropriate for modern coding.
Here's the default implementation:
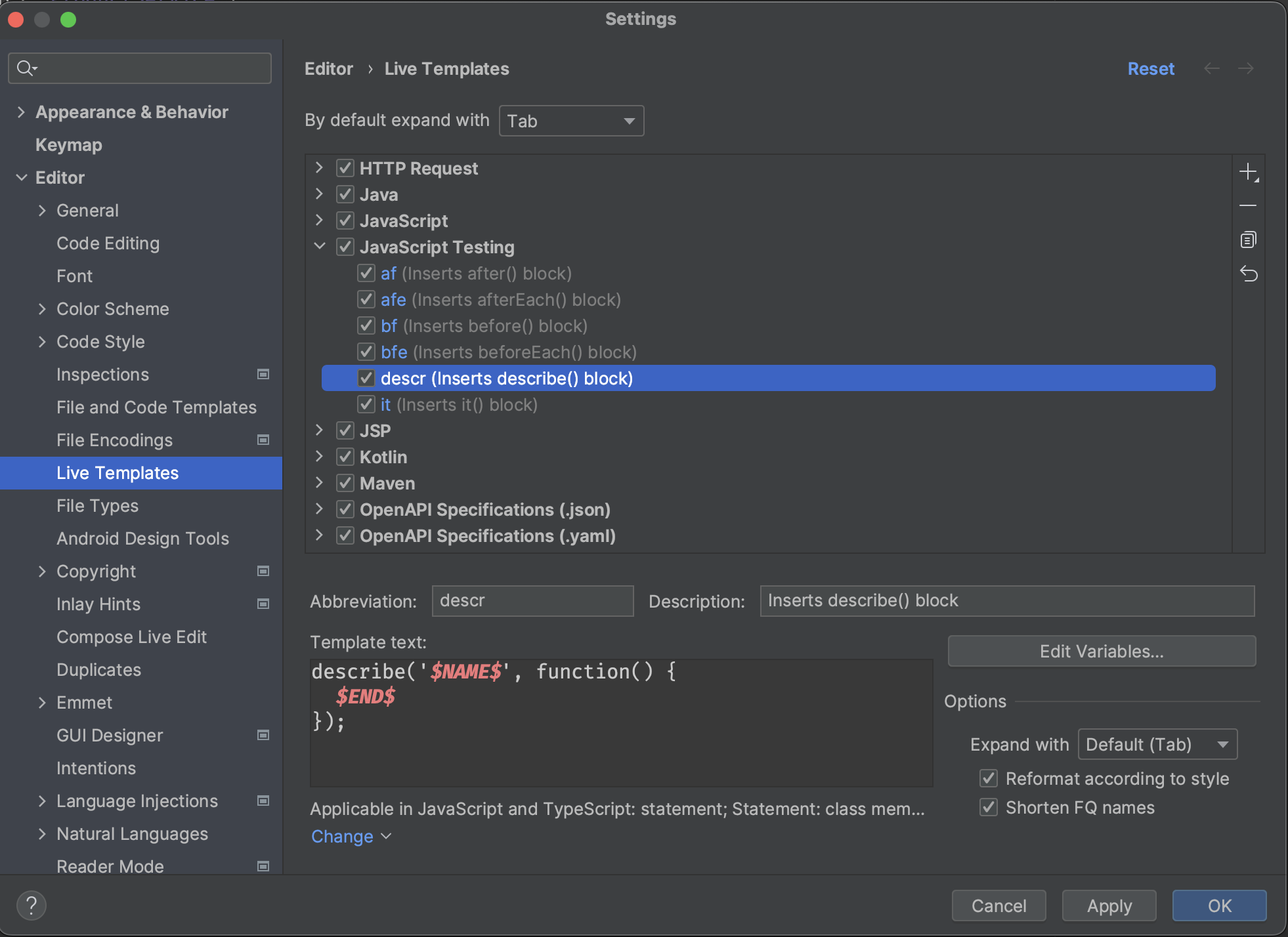
But now that we've seen how to create our own, we also know how to change this one. Just edit the Template text field and hit save!
By the way, did you notice that $END$ variable? Its a very clever way to steer the user to a certain line in the code and simply continue editing there.
This has been a guide to code snippets in IntelliJ, but of course, VSCode has similar functionality as well. More info here